Jpa를 이용하여 게시판 만들어 보기 4
댓글/대댓글
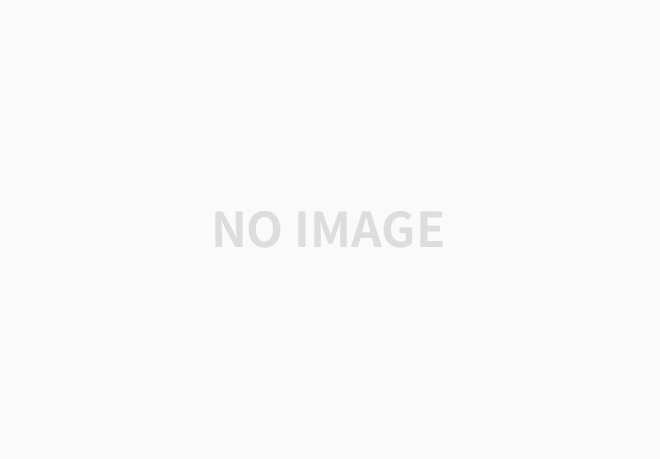
이런 형식으로 댓글과 대댓글을 작성하고 수정할 수 있다.
댓글 엔티티
@Entity
@NoArgsConstructor
@Getter
@Setter
@Table(name = "comment")
public class Comment {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long replyId;
@Column
private Long boardId;
@Column
private Long commentId;
@Column
private String userId;
@Column
private String contents;
@Column
private String userName;
@Column
private int state;
@Column
private LocalDateTime created;
@Column
private LocalDateTime updated;
@Column
private LocalDateTime deleted;
}
replyId 는 모든 댓글과 대댓글의 고유 번호이다.
boardId 는 현재 그 댓글/대댓글을 작성한 게시글의 번호가 저장된다.
commentId 는 기본값을 0으로 저장되고 만약 대댓글인 경우 언떤 댓글의 대댓글인지 알기 위해 대글의 replyId 값이 저장된다.
userId 는 작성한 유저 아이디를 저장한다.
contents 는 작성한 내용
userName 는 작성자의 닉네임
state 는 현 댓글/대댓글의 가시성 상태를 표시한다 1이면 확인할수 있는댓글이고 0이면 확인할수 없는 댓글이다 (삭제 되었을경우)
created 는 작성한 날짜
updated 는 수정 날짜
deleted 는 삭제 날짜
댓글작성
public String create(String contents, Long boardId, HttpServletRequest request, RedirectAttributes redirectAttributes){
HttpSession session = request.getSession();
Users users=(Users) session.getAttribute("userInfo");
String referer = request.getHeader("Referer");
if (users==null){
redirectAttributes.addFlashAttribute("message", "로그인 해주세요");
return "redirect:" + referer;
}
Comment comment = new Comment();
comment.setBoardId(boardId);
comment.setUserId(users.getId());
comment.setContents(contents);
comment.setCreated(LocalDateTime.now());
comment.setState(1);
comment.setUserName(users.getName());
comment.setCommentId(0L);
boolean check =commentService.create(comment);
if (check) {
redirectAttributes.addFlashAttribute("message", "댓글 작성 성공");
} else {
redirectAttributes.addFlashAttribute("message", "댓글 작성 실패");
}
댓글을 작성하는 코드로 세션으로부터 로그인정보(userInfo)를 받아와 현재 로그인했는지 확인한다.
로그인 해야지만 댓글을 작성 할 수 있도록 하였다.
그후 로그인정보를 축출하여 현재 작성자의 정보를 댓글 엔티티에 필요한 정보를 추가하고 작성 내용을 추가하여
commentService.create(comment) 러 댓글 정보를 저장한다.
commentService.create
@Transactional
public boolean create(Comment comment){
try {
commentRepository.save(comment);
return true; // 저장 성공 시 true 반환
} catch (Exception e) {
// 예외 발생 시 false 반환
return false;
}
}
댓글 작성을 db에 전달하여 성공여부를 리턴하여 체크 하도록하였다.
대댓글 작성
public String replyCreate(String contents,Long replyId, Long boardId, HttpServletRequest request, RedirectAttributes redirectAttributes){
HttpSession session = request.getSession();
Users users=(Users) session.getAttribute("userInfo");
String referer = request.getHeader("Referer");
if (users==null){
redirectAttributes.addFlashAttribute("message", "로그인 해주세요");
return "redirect:" + referer;
}
Comment comment = new Comment();
comment.setBoardId(boardId);
comment.setUserId(users.getId());
comment.setContents(contents);
comment.setCreated(LocalDateTime.now());
comment.setState(1);
comment.setUserName(users.getName());
comment.setCommentId(replyId);
boolean check =commentService.create(comment);
if (check) {
redirectAttributes.addFlashAttribute("message", "댓글 작성 성공");
} else {
redirectAttributes.addFlashAttribute("message", "댓글 작성 실패");
}
// 사용자가 요청을 보낸 페이지로 리다이렉트
return "redirect:" + referer;
}
이코드는 댓글 작성 코드와 거의 동일하다 다만 차이가 나는점은 comment.setCommentId(replyId); 이부분이 0이 아니라 어떤 댓글의 대댓글인지 확인할 수 있도록 댓글의 id를 추가한다.
댓글/대댓글 수정
public String replyMdifye(String contents,Long replyId, Long boardId, HttpServletRequest request, RedirectAttributes redirectAttributes){
HttpSession session = request.getSession();
Users users=(Users) session.getAttribute("userInfo");
String referer = request.getHeader("Referer");
if (users==null){
redirectAttributes.addFlashAttribute("message", "로그인 해주세요");
return "redirect:" + referer;
}
Comment comment = commentService.replyMdifye(replyId);
comment.setContents(contents);
comment.setUpdated(LocalDateTime.now());
boolean check =commentService.create(comment);
if (check) {
redirectAttributes.addFlashAttribute("message", "댓글 수정 성공");
} else {
redirectAttributes.addFlashAttribute("message", "댓글 수정 실패");
}
댓글 /대댓글 작성과 마찬가지로 세션으로 부터 유저 정보를 받아온다 그리고 그후
Comment comment = commentService.replyMdifye(replyId); 이걸 통해 현재 수정한 댓글의 정보를 받아 오게 한다.
public Comment replyMdifye(Long replyId) {
return commentRepository.findByReplyId(replyId);
}
아주 간단한 코드로 replyId와 일치하는 댓글을 가져온다는 내용이다.
comment.setContents(contents);
comment.setUpdated(LocalDateTime.now());
그후 코든느 각각 수정된 내용 기입과 현재 시간을 기입하여 수정한 날짜를 기록하여 다시 저장한다.
댓글/대댓글 삭제
public String delete(@RequestParam("replyId")Long replyId,
RedirectAttributes redirectAttributes,HttpServletRequest request) {
HttpSession session = request.getSession();
Users users = (Users) session.getAttribute("userInfo");
String referer = request.getHeader("Referer");
if (users == null) {
redirectAttributes.addFlashAttribute("message", "로그인 해주세요");
return "redirect:" + referer;
}
Comment comment = commentService.replyMdifye(replyId);
comment.setDeleted(LocalDateTime.now());
comment.setState(0);
boolean check = commentService.create(comment);
if (check) {
redirectAttributes.addFlashAttribute("message", "댓글 삭제 성공");
} else {
redirectAttributes.addFlashAttribute("message", "댓글 삭제 실패");
}
return "redirect:" + referer;
}
이것또한 세션에서 유저 정보를 받아오고 댓글 수정할때와 마찬가지로 replyId로 댓글정보를 받아와
comment.setDeleted(LocalDateTime.now());
comment.setState(0);
가시성 상태를 0으로 변경하고 삭제 날짜를 기입하여 다시 저장한다.
게시글 페이지 댓글/대댓글 정보 불러오기
//게시글 확인
@GetMapping("/view")
public String viewPost(
@RequestParam("boardId") Long boardId,
Model model,HttpSession session) {
//보더 아이디로 게시글 내용 조회 하기
Board board = boardService.viewPost(boardId);
//세션의 정보 확인 절차
boolean check=boardService.postCheck(session,board);
//댓글 조회
List<Comment> comments = commentService.commentFind(boardId);
Users users =(Users)session.getAttribute("userInfo");
if (users!=null){
model.addAttribute("userid",users.getId());
}
// 받은 파라미터를 사용한 로직 작성
model.addAttribute("Board", board);
model.addAttribute("check",check);
model.addAttribute("comments",comments);
return "viewPost"; // viewPost.jsp로 데이터 전달
}
List<Comment> comments = commentService.commentFind(boardId);
boardId 를 이용하여 댓글 정보를 불러온다.
commentFind
public List<Comment> commentFind(Long boardId){
//댓글조회
List<Comment> comments = commentRepository.findAllByBoardIdAndCommentIdAndState(boardId,0L,1);
ArrayList<Comment> list =new ArrayList<>();
for(int i=0; i<comments.size();i++){
Comment comment = comments.get(i);
list.add(comment);
//대대글 조회
List<Comment> commentReply = commentRepository.findAllByBoardIdAndCommentIdAndState(boardId,comment.getReplyId(),1);
if (commentReply != null) {
for(int j=0; j<commentReply.size();j++){
list.add(commentReply.get(j));
}}
}
return list;
}
댓글/대댓글을 조회할때 현재 게시글에서 가시성 상태가 1이고 commentId 0인 댓글을 일단 모두 불러온다
그리고 불러온 댓글에 대댓글이 존재하는지 확인하기 위해
for문을 이용하여 하나하나 탐색하는데 이때 for문이 돌때마다 현재 댓글은 리스트에 저장되고 그후
List<Comment> commentReply = commentRepository.findAllByBoardIdAndCommentIdAndState(boardId,comment.getReplyId(),1);
현재 댓글의 아이디와 가시성1 인걸을 모두다 조회 하여 그 댓글에 달린 모든 대댓글을 불러와 값들을 리스트에 저장한다.
이 값들을 저장한 리스트가 바로 그 게시글에 작성된 댓글과 대댓글들이다.
'자바 공부 > 스프링공부' 카테고리의 다른 글
스프링부트 Scheduled 사용법 (1) | 2024.09.17 |
---|---|
스프링부트 메이븐 - MyBatis 사용법 (2) | 2024.09.04 |
Jpa를 이용하여 게시판 만들어 보기 3 (0) | 2024.08.19 |
Jpa를 이용하여 게시판 만들어 보기 2 (0) | 2024.08.08 |
jpa를 이용하여 게시판 만들어 보기 1 (0) | 2024.08.07 |