이메일 인증
- 앞서 스프링에서 이메일 인증을 하기위해 구글 이메일 설정및 앱 비밀번호 생성하는 부분은 생략하겠다.
1. 의존성 추가
이메일 기능을 사용을 위한 의존성 추
implementation 'org.springframework.boot:spring-boot-starter-mail'
2.application.yml 설정
spring:
mail:
host: smtp.gmail.com
port: 587
username: ${MAIL_USERNAME}
password: ${MAIL_PASSWORD}
properties:
mail:
smtp:
auth: true
starttls:
enable: true
timeout: 5000
connectiontimeout: 5000
여기서 MAIL_USERNAME 은 설정한 본인의 구글 이메일 주소를 기입해야한다.
MAIL_PASSWORD 는 구글에서 설정된 보인의 앱 비밀번호를 입력하면된다.
3.MailService 구현
@RequiredArgsConstructor
@Service
public class MailService {
private final JavaMailSender javaMailSender;
@Value("${MAIL_USERNAME}")
private String senderEmail;
private String verificationCode;
private void createVerificationCode() {
Random random = new Random();
StringBuilder key = new StringBuilder();
for (int i = 0; i < 8; i++) {
int idx = random.nextInt(3);
switch (idx) {
case 0 -> key.append((char) (random.nextInt(26) + 97));
case 1 -> key.append((char) (random.nextInt(26) + 65));
case 2 -> key.append(random.nextInt(9));
}
}
this.verificationCode = key.toString();
}
private MimeMessage createMail(String recipientEmail) {
createVerificationCode();
MimeMessage message = javaMailSender.createMimeMessage();
try {
message.setFrom(senderEmail);
message.setRecipients(MimeMessage.RecipientType.TO, recipientEmail);
message.setSubject("이메일 인증 코드");
String htmlContent = String.format("""
<html>
<body>
<div style="font-family: Arial, sans-serif; padding: 20px;">
<div style="background-color: #4CAF50; color: white; padding: 10px; text-align: center;">
<h2>이메일 인증</h2>
</div>
<div style="background-color: #f4f4f4; padding: 20px; border-radius: 5px;">
<p>안녕하세요,</p>
<p>귀하의 이메일 인증 코드는 다음과 같습니다:</p>
<p style="font-size: 24px; font-weight: bold; color: #4CAF50; letter-spacing: 5px;">%s</p>
<p>이 코드를 입력하여 이메일 인증을 완료해 주세요.</p>
</div>
<div style="margin-top: 20px; font-size: 12px; color: #777; text-align: center;">
<p>본 메일은 발신 전용입니다. 문의사항이 있으시면 고객센터로 연락해 주세요.</p>
<p>© 2025 Your Company Name. All rights reserved.</p>
</div>
</div>
</body>
</html>
""", verificationCode);
message.setText(htmlContent, "UTF-8", "html");
} catch (MessagingException e) {
throw new RuntimeException("메일 생성 중 오류 발생", e);
}
return message;
}
public String sendMail(String recipientEmail) {
MimeMessage message = createMail(recipientEmail);
javaMailSender.send(message);
return verificationCode;
}
}
처음 sendMail 이 실행되고 sendMail 안에서 CreateMail이 실행되어 메일을 전송하는 내부를 만들어준다.
나는 인증번호가 필요하기에 createVerificationCode 이 메소드를 통해 인증 번호를 랜덤으로 만들어 전송하였다.
4.Controller 구현
@RestController
@RequiredArgsConstructor
public class MailController {
private final MailService mailService;
@PostMapping("/send-email")
public ResponseEntity<String> sendVerificationEmail(@RequestParam String email) {
try {
mailService.sendMail(email);
return ResponseEntity.ok("인증 메일이 발송되었습니다.");
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body("메일 발송 실패: " + e.getMessage());
}
}
}
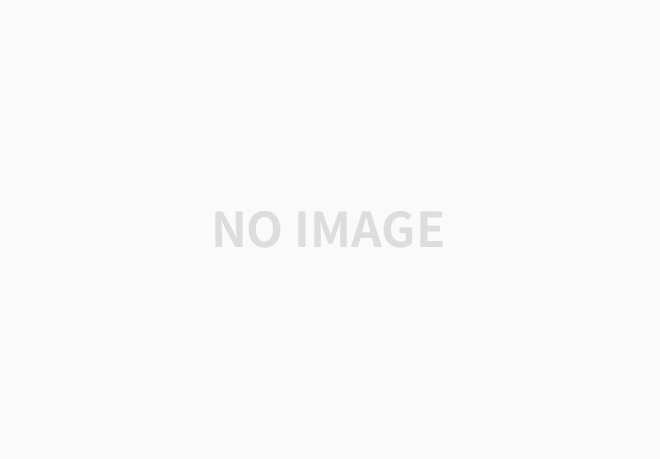
반응형
'자바 공부 > 스프링공부' 카테고리의 다른 글
Spring ExceptionHandler을 사용한 예외처리 (0) | 2025.03.16 |
---|---|
스프링 Junit5 와 Mockito를 이용한 단위 테스트 (0) | 2025.02.18 |
스프링부트 시큐리티(Security) 6 config 작성방법 (1) | 2024.09.27 |
스프링 부트 웹소켓 Stomp 사용법 (0) | 2024.09.19 |
스프링부트 Scheduled 사용법 (1) | 2024.09.17 |